Receive Captured Images
The application has to be set-up to receive captures, to be able to acquire the images from the connected camera after capture.
After opening the camera, image receiving can be enabled, as follows:
camera.EnableImageReceiving(true);
camera.EnableImageReceiving(true);
Now, a capture can be triggered:
camera.TriggerCapture();
camera.TriggerCapture();
To receive the image, use the WaitForImage
method, as follows:
P1::CameraSdk::IIQImageFile imageFile = camera.WaitForImage(60000);
IIQImageFile imageFile = camera.WaitForImage(timeoutMs: 10000);
The WaitForImage
method is a blocking call, which will listen for captures as long as the application is running, or until the specified time has passed with no capture received.
The timeout can be specified as a parameter of the method. If the timeout is reached, the CameraSDK will throw an exception (in C++), or return a null
value (in C#) (see Error Handling and Logging). A timeout-value of “0” means “wait forever” – this is the default, if no value is specified.
Caution
After an image has been received, the CameraSDK “owns” the memory until a destructor is used on the imageFile
object. See Memory Management for more.
Note
The WaitForImage
method returns only one image for each call. Thus, every time an image is captured on the camera, the WaitForImage
method has to be called to receive the image.
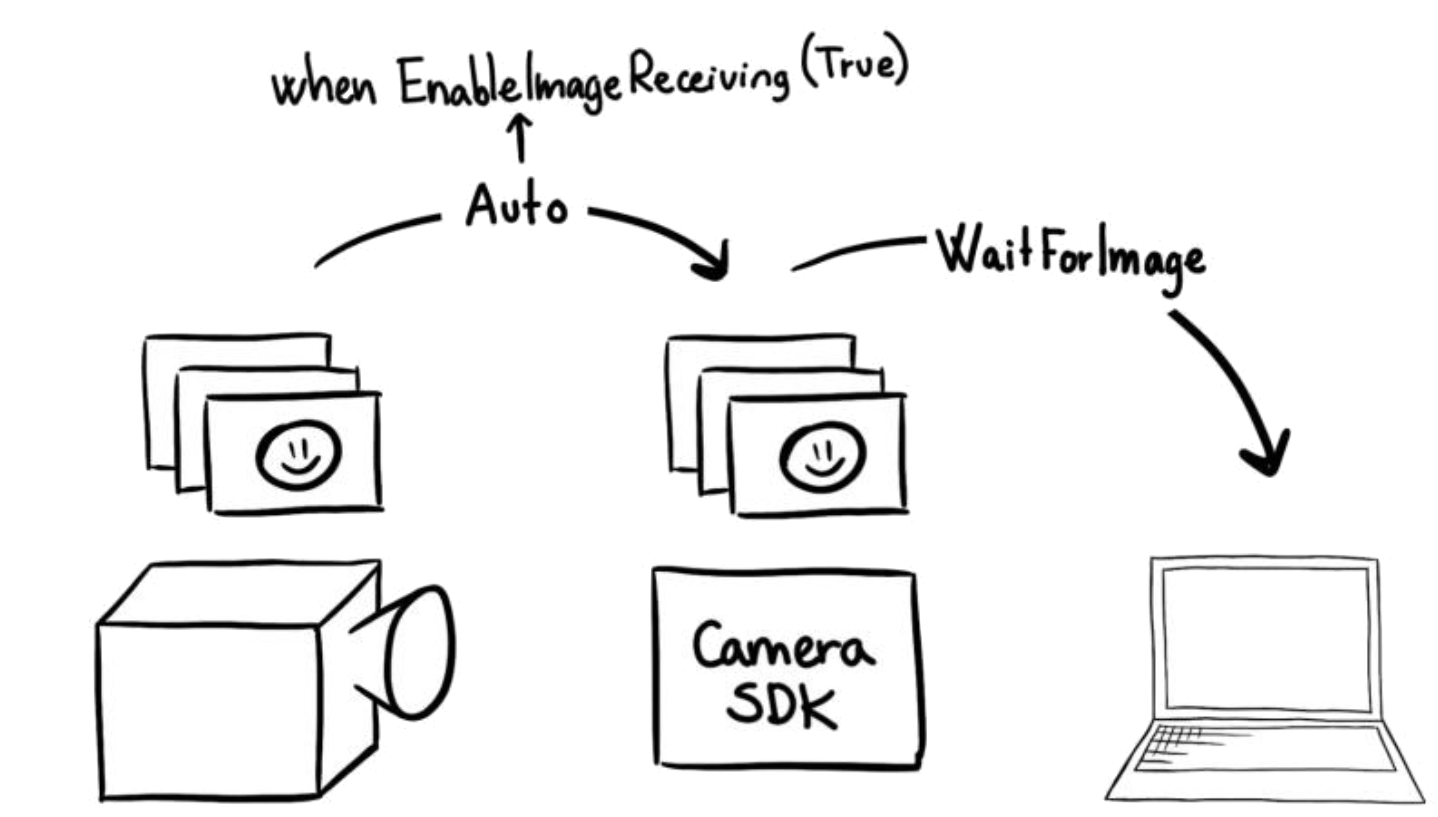
When transferring images from the camera to the application, there are two separate image queues. The queue of images from the camera to the CameraSDK is auto-handled by the CameraSDK, when EnableImageReceiving
is set to “true
”. The WaitForImage
method is waiting on images from the CameraSDK’s queue – not the camera’s internal queue – and returns the next image from the CameraSDK’s queue each time it is invoked, until the CameraSDK’s queue is empty.
Note
The queue of images in the camera can be investigated using the UnsavedImages
property. The CameraSDK’s queue can only be investigated by using the WaitForImage
method; if no images are returned, no images are in the CameraSDK’s queue.
All threads blocked by a WaitForImage-call are released if the camera is disconnected. The waiting thread can also be forcefully released by another thread, as defined in the following example:
camera.WakeUpWaitingImageThreads();
camera.WakeUpWaitingImageThreads();
Caution
The WakeUpWaitingImageThreads
call releases blocked threads, held in the camera’s WaitForImage
and WaitForLiveView
(see LiveView) methods.