Memory Management
When initiating the application and the CameraSDK, RAM is allocated from the Host computer.
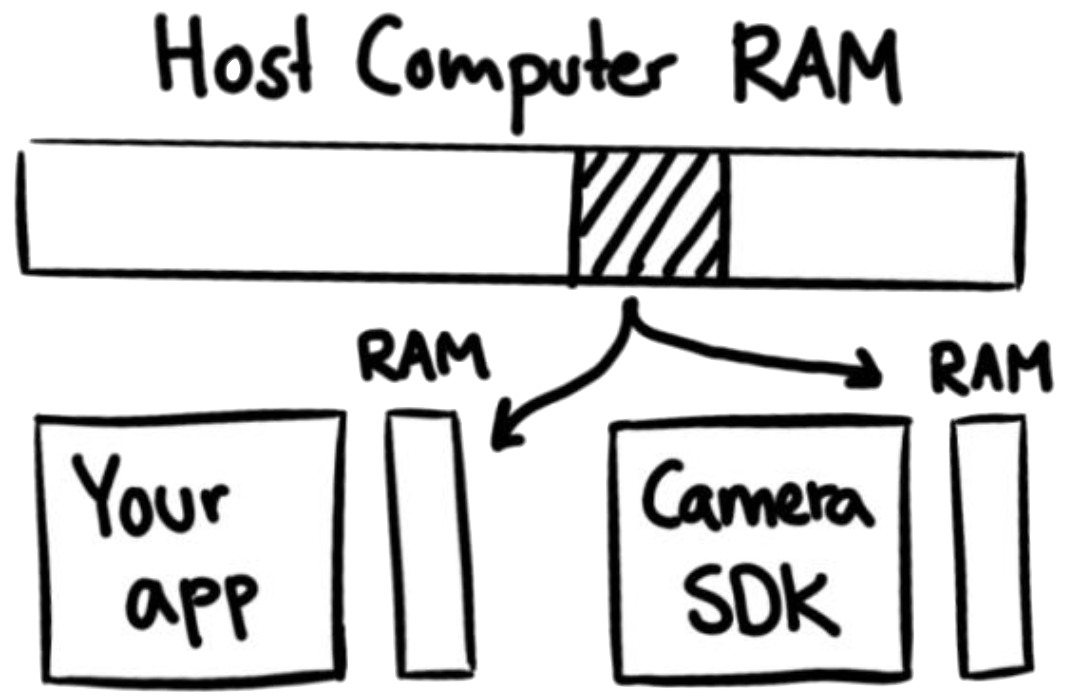
Currently, the CameraSDK handles images in a way where the user gets the entire image (when requesting it). In C++, the image is handed to the application in a shared_ptr
-object (shared pointer). In C#, we use the dispose pattern.
C++ |
C# |
---|---|
|
|
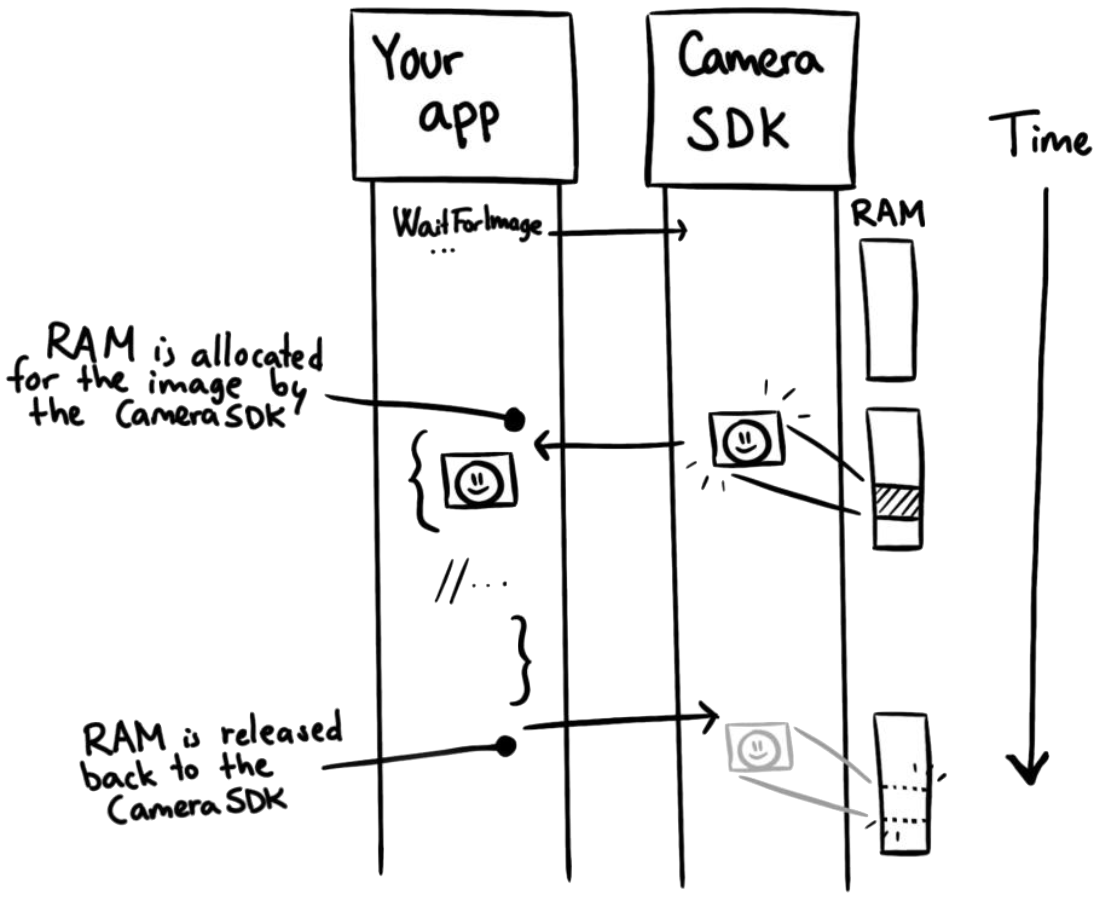
This means that the application “borrows” RAM from the CameraSDK, while handling the image. When the shared_ptr
-object – the image - goes out of scope, the shared_ptr
object is destroyed. Upon destruction, the “borrowed” RAM is then released back to the CameraSDK.
Note
In C++, the shared_ptr
objects are automatically destroyed upon going out of scope.
In C#, the “using
” statement should be used to handle the object. By using the “using
”-statement, the object is disposed immediately when it goes out of scope:
using (var image = camera.WaitForImage())
{
// do something with image here
} // image is disposed here
Note
Manually disposing the image by using image.Dispose
is possible, however, not recommended. Manually disposing can cause issues, e.g. if an exception is thrown while executing the code, causing an early exit.
To learn more about how to use objects that utilize the dispose pattern, see Microsoft documentation on the subject: Using objects that implement IDisposable
If you need to retain the image, or parts of the image (e.g. a preview), it must be copied to another place. Since the memory used by the shared_ptr
(or an IDisposable
) object should be returned to the CameraSDK as soon as possible. Failing to do so, can cause the CameraSDK to eventually run out of memory.
See more details on handling memory in C# in C# Memory Handling.