A Thread-model for the CameraSDK
In the code samples, a single thread has been used for the sake of simplicity. In reality, we recommended a multi-threaded approach. In this section, we suggest a thread-model for working with the CameraSDK.
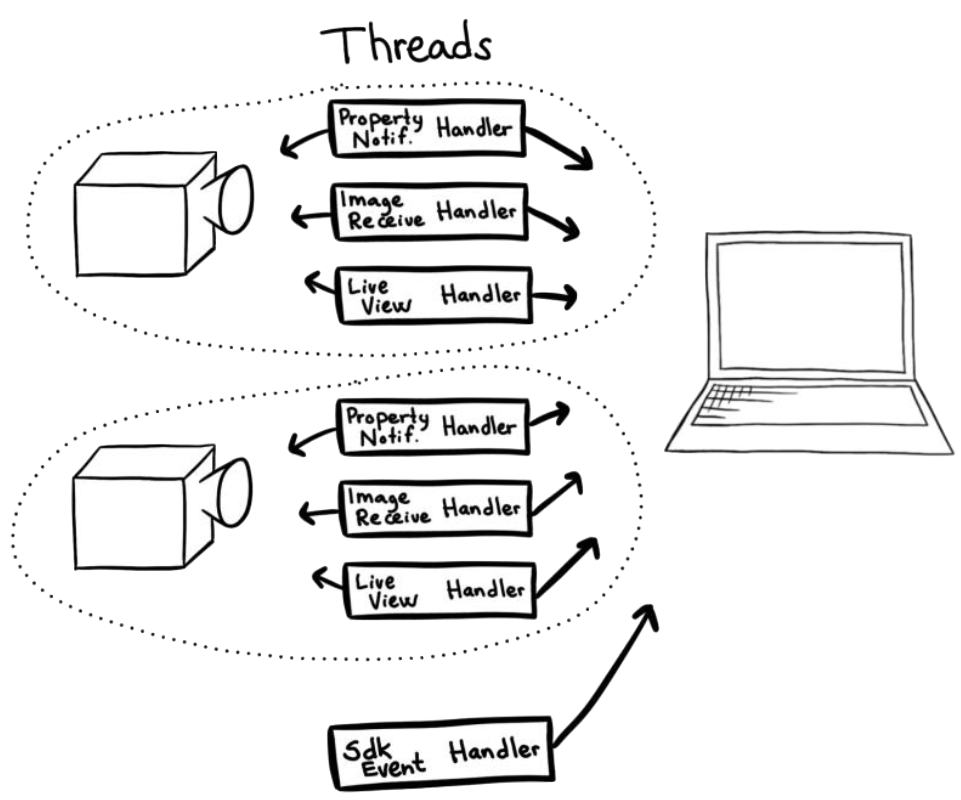
One possible thread-model is as follows: Create one thread to handle SdkEvents
regardless of connected cameras (this is used to e.g. notice, when a camera is available to be opened). Additionally, create 3 threads per connected camera:
One to handle notifications (WaitForNotification – this includes both PropertyEvents and CameraEvents),
one to handle the reception of images (WaitForImage), and
one to handle LiveView (WaitForLiveView).
Implementing this thread-model allows for the program to handle several blocking calls simultaneously, independently from each other. Thus, the threads do not have to wait on processes that may be slower to finish before they can execute the tasks (e.g. receiving an image before being able to capture a new one).
When using multiple threads, you should implement clean-up procedures as well. These should close/release threads that are no longer needed.
You can use the following method to release waiting threads held in this camera’s WaitForImage
and WaitForLiveView
methods:
camera.WakeUpWaitingImageThreads();
camera.WakeUpWaitingImageThreads();
To release the notification-thread, you use the following methods:
// Force the pending thread in WaitForNotification() to be released
listener.WakeUpWaitingThread();
// Wait here until listen thread has terminated gracefully
listenThread.join();
// Force the pending thread in WaitForNotification() to be released
listener.WakeUpWaitingThread();
// Wait here until listen thread has terminated gracefully
listenThread.Join();
Note
Closing the camera will release all waiting threads for that camera, causing an implicit call to WakeUpWaitingImageThreads
.