Receive Captured Images
The SDK can be set-up to receive captures, to be able to acquire the images from the connected camera right after a capture. To enable receiving images you subscribe to the Full Image subscription.
When you receive full images, you will get the entire IIQ file in a memory buffer. Write the buffer to a file, and that would be valid .iiq
Phase One Raw file, that can be opened by Capture One and iX Process.
After subscribing you will begin to receive notifications about new image captures. These notification objects includes the actual image data buffer. Such notifications are of the sub-category Image Receiving, for transfer progress reporting. However, the image buffer is included only in the CameraImageReady
event.
To enable image receiving, first activate the Full Image subscription on the camera:
camera.Subscriptions()->FullImages()->Subscribe();
The moment you subscribe to Full Image, the camera will regard your application (running CameraSDK), as a new storage option. New captures will pushed to your host, just like they will be pushed to any present memory card(s).
This means you must be ready to catch the incoming notifications, when the subscription is enabled. To receive the image, use the Listener
object, introduced in the Begin Listening for Notifications section:
P1::CameraSdk::Listener listener;
listener.EnableNotification(camera, P1::CameraSdk::EventType::CameraImageReady);
std::shared_ptr<const P1::CameraSdk::INotificationEvent> notification = listener.WaitForNotification(60000);
std::shared_ptr<const P1::CameraSdk::IFullImage> imageFile = notification->FullImage();
With a listener setup, we can trigger a capture on the camera:
camera.TriggerCapture();
camera.TriggerCapture();
Image Queues
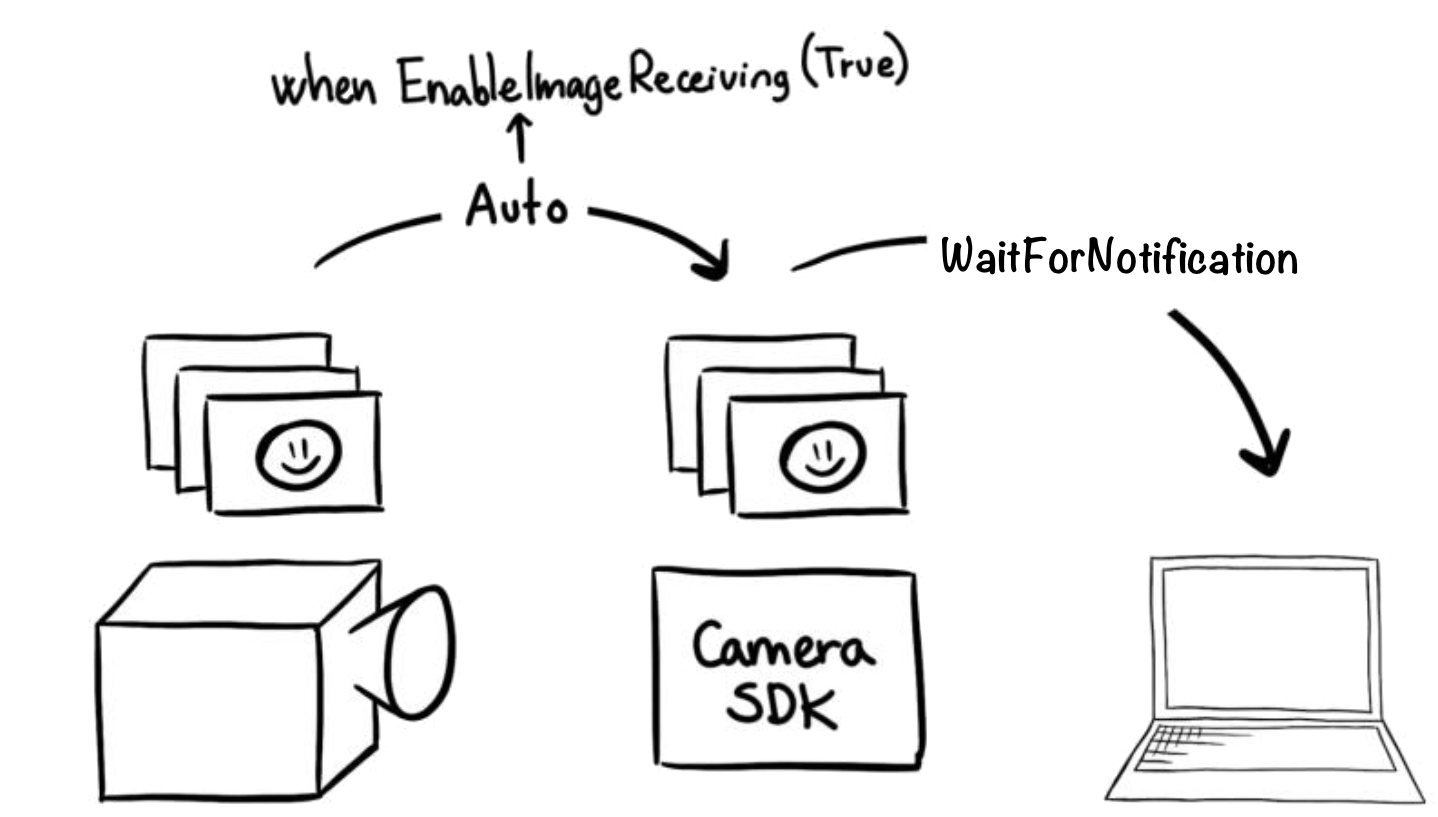
When transferring images from the camera to your application, there are two separate image queues. There is the queue of images from the camera to the CameraSDK. This queue is part of the regular notification system, and incoming images are discarded if no one is listening.
Inside the camera there is a another queue, where images are waiting until they are stored and/or, transferred to your host application. When the full image subscription is active, the camera will push all images in its queue, to the notification queue in the CameraSDK.
Note
The queue of images in the camera can be investigated using the UnsavedImages
property. The CameraSDK’s internal queue can only be investigated by dequeueing notifications on a Listener
; if no CameraImageReady
notifications are returned, no images are in the notification queue.
Tracking Transfer Progress
If you are working with the camera on a slow link, like Wifi or USB2, you probably want to follow the transfer progress.
All full images (IIQ files) are delivered via the CameraImageReady
notification. This notification is posted after the entire IIQ file is transferred. However, before that happens you can receive two kinds of transfer status notification: ImageTransferBegin
and ImageTransferProgress
.
Image transfer Begin is posted when a new image transfer is pushed by the camera, indicating that 0% of the transfer is completed.
Image Transfer Progress is posted at every percent increment of the transfer progress. A rate limitation ensures you will never receive more than 10 progress notifications per seconds. This means if the connection link is fast enough, you will see percentage increment with more 1, at each posted notification.
To receive transfer progress notifications, you need to setup a Listener
to receive the notifications. Then you subscribe to Full Image:
Listener listener;
listener.EnableNotification(camera, EventType::ImageTransferBegin);
listener.EnableNotification(camera, EventType::ImageTransferProgress);
listener.EnableNotification(camera, EventType::CameraImageReady);
camera.Subscription()->FullImageSubscription()->Subscribe();
camera.TriggerCapture();
std::shared_ptr<const INotificationEvent const> notification;
do
{
notification = listener.WaitForNotification();
std::cout << notification->TransferProgressPercent() << "\n";
}
while(notification.Type().id != EventType::CameraImageReady.id);
All Image Receiving category notifications has a TransferProgressPercent()
method, that return the current transfer progress.
The CameraImageReady
will be posted, instead of the final 100% ImageTransferProgress
, and only this notification will include the actual IIQ file.