Notifications
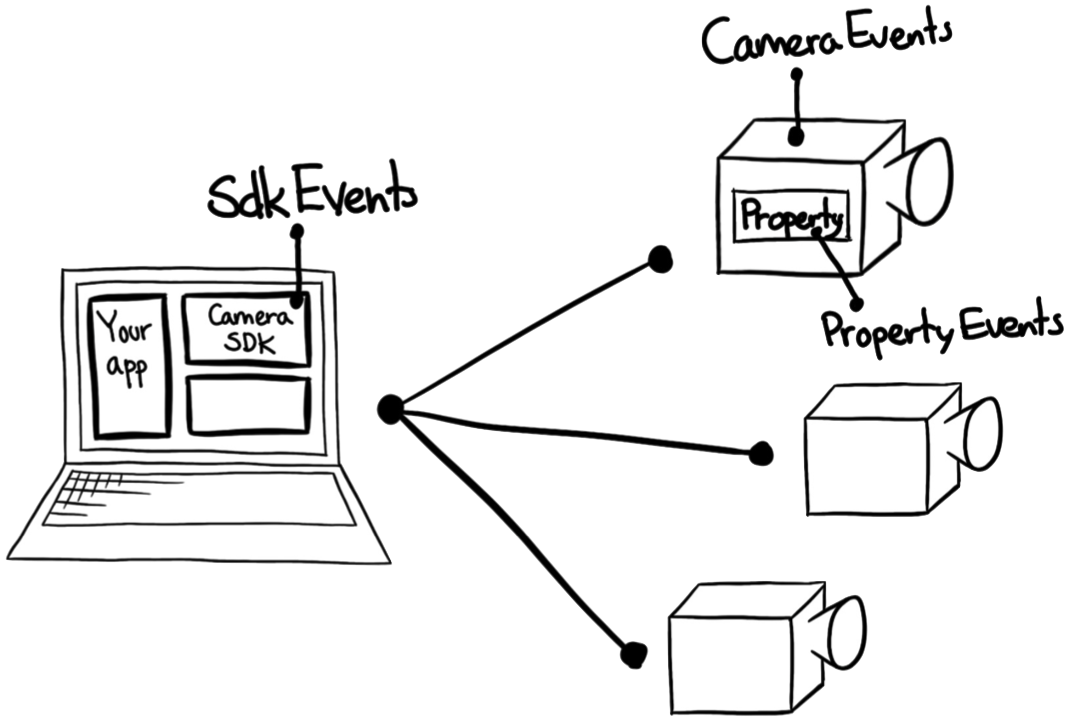
Events happening in the camera, can generate notifications that are received on the Host computer. These can be of various categories, each telling something about what payload data you can expect to be delivered with the notification.
Note
The notification API has changed from CameraSDK release version 2.0 to 3.0! If you previously worked with the notifications API’s in version 2.0, you will find that the API has completely changed in 3.0. And that these are breaking changes!
All notifications, have to be enabled for the events to generate notifications. These notifications can be enabled or disabled at any time.
To listen for notifications, create an instance of the Listener
class:
P1::CameraSdk::Listener listener;
Listener listener = new Listener();
The Listener
class acts as a notification central, where the notifications from enabled events arrive. You receive the incoming notifications by calling the blocking method: WaitForNotification
on the Listener
object. Since it is blocking it should be called from a separate thread (see Begin Listening for Notifications).
A thread listening for notifications should be offloaded by using other threads to handle “reactions” to the notifications (see A Thread-model for the CameraSDK). In case the no thread is blocked inside WaitForNotification
, notifications are queued (FIFO). Next call to WaitForNotification
will return the next waiting notification, in order. This way, notifications are not lost.
Multiple notifications can be enabled per Listener
instance. Furthermore, multiple Listener
instances can be created, as needed. Each instance of Listener
acts independently of other Listener
instances.
You can create multiple Listener
objects, that are setup to receive the notifications originating from the same events. In this case, each listener will actually receive the same notification object. That is why notification objects are delivered inside a std::shared_ptr
object in C++.
Event Categories
All notification types belongs to a category. These denote the area the notification belong to, and define what payload data is included in the notification object.
The next section describes the categories in further detail.
SDK Events
The SdkEvent
category describes a change at the CameraSDK-level. For instance, if the CameraSDK discovers a USB connected camera, a notification of the type “SdkEvent
” is generated.
An example of listening for the event of a camera being found (CameraFound
) is shown below:
listener.EnableNotification(P1::CameraSdk::SdkEventType::SdkCameraFound);
listener.EnableNotification(SdkEventId.CameraFound);
SDK Events will tell you when USB cameras are discovered and when they disappear. The CameraFound
notification and its opposite CameraRemoved
, informs you about the visible USB cameras. It does not matter whether you are connected (camera is opened), or not.
Caution
TCP/IP cameras are not discovered. You will not receive any found or removed notifications from TCP/IP cameras.
Camera Events
The CameraEvent
category of notifications, reflect events in a connected (and opened) camera. For example, if the camera delivers an image to the SDK host. This can be seen in the example below:
listener.EnableNotification(camera, P1::CameraSdk::EventType::CameraImageReady);
listener.EnableNotification(camera, CameraEventId.ImageReady);
The Camera event category is further divided into 4 sub-categories: image receiving, connection, LiveView & property lifetime. The next few sections will examine these sub-categories in more detail.
Image Receiving
These are camera events that provide progress reporting on the image transfer between camera and host. When the camera starts transferring an image, the ImageTransferBegin
event is received. At rate-limited intervals the SDK will provide the TransferProgress
event, until the entire image is present in SDK memory. Then ImageReady
is received, and the image is included inside that notification object.
Connection
The Disconnected
camera event indicates that the connection (USB / TCP) is lost. This is either by intention or as a result of a link error. You will receive the Disconnect
event after you call Close
on the camera. Similarly, you will receive the same event if the USB or network cable has suddenly been unplugged. The Disconnect
event itself cannot tell you with scenario it comes from!
Caution
TCP socket on certain platforms are very forgiving, and will try to keep the TCP connection open for several minutes.
If you are on a poor quality Wifi, this is probably a desired behavior. On the other hand, if you are on cabled and switched ethernet, then even an unplugged cable is not enough to interrupt the established TCP connection.
LiveView
The LiveView related camera events will indicate when LiveView is started or stopped. Also, the NewLiveViewImage
event type provides you with the new LiveView image, inside the notification object.
If you are working with an IQ4 digital back, starting LiveView on the cameras display will not trigger any LiveView notifications in the SDK. LiveView notifications received in the SDK, are when LiveView is used by the SDK itself.
Property Lifetime
These are camera events that advertises about camera properties that appear or disappear. The camera can change mode, which might trigger new properties to become available. Likewise, changing a mode on the camera might cause some properties to disappear.
Property Events
A PropertyEvent
-type notification is triggered upon the changing of a value in a property, such as the “aperture” being set to “5.6”.
To receive notifications about property events, notifications have to be enabled for the desired property (e.g. “aperture” or “ISO”).
When enabling notifications for a property, a notification arrives when the property changes its value.
An example of how to listen for a change in the “aperture”-property can be seen below:
listener.EnableNotification(camera, 1003); // Aperture
listener.EnableNotification(camera, 1003); // Aperture
Note
An attempt to set the value to its current value (e.g. “5.6” –> “5.6”) will not generate a notification.
Specification Change Events
By default a property event denote a change in the property’s value. However, the property’s specification (PropertySpec
) might also change. These changes could be the read only state or a change in the list of valid values (the value Map).
Just as you can listen for value changes, you can likewise listen for specification changes - that does not change the property’s value.
Arriving specification change notifications, are of the SpecificationChange
type, where as property value change notification are of type Property
.
To enable notifications for specification changes, you use the same EnableNotification
method, but provide a 3rd argument:
listener.EnableNotification(camera, 1003, true); // 1003 is Aperture
listener.EnableNotification(camera, 1003, isSpecificationChange: true); // 1003 is Aperture
This 3rd and optional argument defaults to false
. If you explicitly set it to true
you will receive only specification state changes for the given property id and camera.
You can enable both property value and specification change notifications, with two calls the method - one for each type. They can exist independently from each other.
Similarly, you disable specification changes by calling DisableNotification
and providing a 3rd argument set to true
:
listener.DisableNotification(camera, 1003, true); // 1003 is Aperture
listener.DisableNotification(camera, 1003, isSpecificationChange: true); // 1003 is Aperture
Begin Listening for Notifications
When notifications have been enabled for the desired events, the listening for notifications can begin. A thread will have to continuously listen for the events, regardless of event type. This thread executes the WaitForNotification
method, as can be seen below:
void listenRoutine(P1::CameraSdk::Listener listener)
{
std::shared_ptr<P1::CameraSdk::INotificationEvent const> arrivedNotification;
while (true)
{
arrivedNotification = listener.WaitForNotification();
if ( ! arrivedNotification)
{
std::cout << "Signalled wake-up!\n";
break;
}
else
{
std::cout << "Notification received\n";
}
if (arrivedNotification->Type().id == P1::CameraSdk::SdkEventType::SdkCameraFound.id)
{
std::cout << "Camera found\n";
break;
}
// Handle other notification types here...
else
{
std::cout << "Got unknown:" << arrivedNotification->Type().name << std::endl;
}
}
}
var listenThread = new Thread(() => {
while (true)
{
NotificationEvent? notification = listener.WaitForNotification();
if (notification.HasValue == false)
{
Console.WriteLine("Signalled wake-up!");
break;
}
if (notification.Value.SdkEventId == SdkEventId.CameraFound)
{
Console.WriteLine("Camera Found");
break;
}
// Handle other notification types here...
else if (notification.Value.PropertyId == apertureId)
{
Console.WriteLine("Aperture changed");
break;
}
else
{
Console.WriteLine("Got unexpected: {0}", notification);
break;
}
}
});
listenThread.Start();
Output: (if the notification was not otherwise handled):
Got unexpected: NotificationEvent (SdkEvent): CameraRemoved
Stop Listening for Notifications
Upon terminating the application, the listening for notifications should terminate as well. To accomplish this, the CameraSDK has a function to release the thread listening for notifications in the specific Listener
, as follows:
// Force the pending thread in WaitForNotification() to be released
listener.WakeUpWaitingThread();
// Wait here until listen thread has terminated gracefully
listenThread.join();
// Force the pending thread in WaitForNotification() to be released
listener.WakeUpWaitingThread();
// Wait here until listen thread has terminated gracefully
listenThread.Join();
Notification Payloads
Each notification type can deliver optional payloads or context data. The NotificationEvent
object defines members that are populated only for specific notification and event types. These members provide contextual information about the notification.
The table below list the available payload members of NotificationEvent
and the conditions that must be met, for them to be populated with meaningful values:
Member Field |
Description |
Condition |
---|---|---|
ImageId |
The image id of the image this event relates to |
All Image Receiving sub-category notifications |
CameraId |
The id of the camera that the notification relates to |
All Camera category notifications |
PropertyId |
The Id of the property that is changed |
All |
BasicImageInfo |
The BasicImageInfo object included in the notification |
Present only for notifications of
|
Preview |
The Preview image object included in the notification |
Present only for notifications of
|
Tile |
The Tile image object included in the notification |
Present only for notifications of
|
LiveView |
The LiveView image object included in the notification |
Present only for notifications of
|
FullImage |
The FullImage IIQ file included in the notification |
Present only for notifications of
|
StoredImageHandle |
The handle to the in camera image |
Present for |
CameraInfo |
A small struct describing the cameras connection type, IP, serial, etc. |
|
TransferProgressPercent |
A progress status for image transfers, in percent. |
CameraEvent category events,
where the sub-category is
|
ImageFilename |
The filename of the image that was transferred |
Any of the Transfer sub-category notifications |
Catch All Listeners
The Listener
class has a special catch all state, that enables receiving every single notification posted by the SDK, without the need for calling EnableNotification
for every notification first.
Notifications are received for all notification types, for every event, across all opened cameras. Also, notifications will be enabled for properties that are not yet visible, (due to the cameras state).
If you enable the catch all state on a Listener
before you open the first camera, you will receive the (CameraEvent type) notification CameraEventPropertyAdded
, when you open any cameras.
To enable catch all on a Listener
instance:
listener.EnableCatchAllNotifications();
listener.EnableCatchAllNotifications();
Disable Catch All State
The catch all state can be disabled at any time, by calling:
listener.DisableCatchAllNotifications();
listener.DisableCatchAllNotifications();
This effectively deactivates the delivery of all notification types to the Listener
instance. The instance’s state is returned to normal, where only enabled notifications will be delivered. (The ones enabled by calling EnableNotification
.)
If Listener
already has any explicitly enabled notifications, these will be preserved. Thus, these are not affected by disabling the catch all state.
Warning
Be aware that disabling the catch all state, does not clear the listeners queue of incoming notifications. Calls to WaitForNotification
might return notifications queued up before the call to DisableCatchAllNotifications
.